free in C
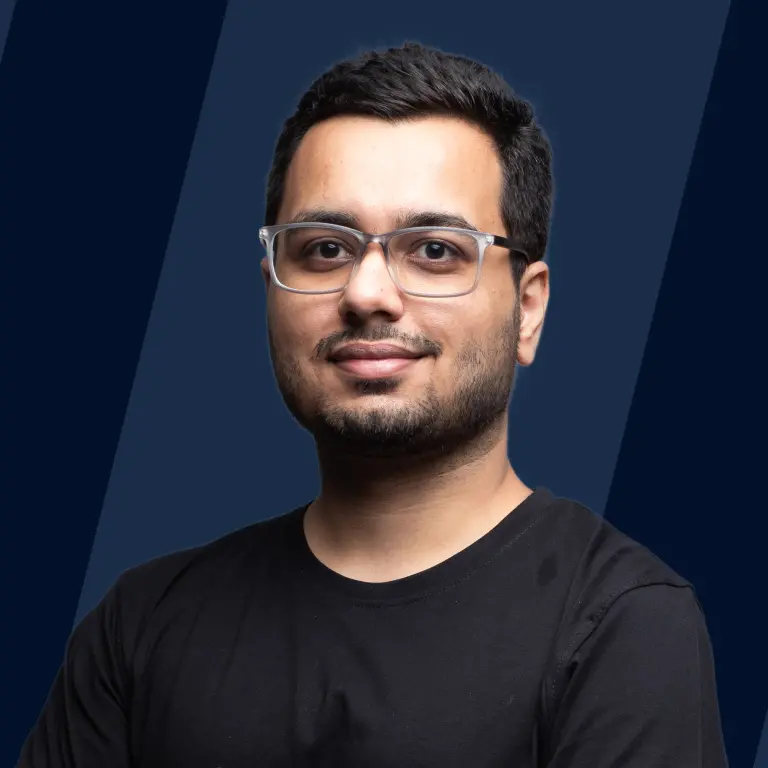
Overview
free in C is a library function present in stdlib.h header file and is used to de-allocate or free up the space allocated by the functions like malloc() and calloc(). It takes only one argument, i.e., the pointer pointing to the memory location to be de-allocated.
Syntax of free in C
- General Syntax in C language:
- free in C is declared in <stdlib.h> header file as:
Parameters of free in C
free in C takes only one parameter.
- ptr: It is a pointer/reference to the memory block that has to be de-allocated. The memory is allocated using the library functions like malloc(), calloc(), or realloc(). Nothing happens if a null pointer is used as an argument.
Return Value of free in C
free in C doesn't return any value. It has a return type of void.
Example of free in C
Deallocating an integer memory block using free in C:
Output:
Explanation: In this program, we have used the free() function to de-allocate the integer memory block allocated using the malloc() function. We have de-allocated the memory pointed by the ptr pointer variable during the run-time of the program.
The below image demonstrates the program flow. First, we have created an integer memory block using the malloc() function and stored the return value of malloc() function, i.e., the address of the integer block into a pointer variable ptr (let the address be 1000). We have stored an integer value of 5 in the integer block using the ptr variable. Next, we have used the free(ptr); statement that deallocates the integer memory block but ptr still contains the address 1000, so it is acting as a dangling pointer and pointing to a garbage value. Lastly, we assign NULL to the ptr variable which helps to avoid the dangling pointer situation.
Exceptions of free in C
- The behavior is not-defined/unknown, if ptr address does not match the value that was returned by malloc(), calloc(), or realloc(),
- The behavior is not-defined if ptr refers to an already de-allocated memory block, and no further calls to malloc(), calloc(), or realloc() produced a pointer equal to ptr.
What is free in C?
free in C is a library function defined in the <stdlib.h> header file which is used to de-allocate the memory blocks. It takes only one argument, i.e., the pointer containing the address of the memory location to be de-allocated. It helps in the reuse of the memory, hence making our program more efficient. As the memory is not de-allocated on its own, so we have to use the free() function to de-allocate the memory during the run-time of a program.
When we de-allocate a block of memory using the free() function, it creates a dangling pointer where the pointer contains a garbage address value. If an access is made using the pointer ptr after free(ptr); statement execution, the behavior is undefined, unless another allocation of memory happened to the pointer ptr. Also, if we don't free up the dynamic memory using the free() function until the program execution finishes, it can lead to memory leaks.
Interesting Fact: realloc(ptr, 0) statement is the same as free(ptr).
More Examples
- C Program for de-allocating a memory block using the free() function allocated using the malloc() function:
Output:
(output garbage values are compiler-dependent)
Explanation: In this program, we have used the free() function to de-allocate a memory block allocated using the malloc() function. We have de-allocated the memory pointed by p character pointer variables during the run-time of the program.
- C Program for de-allocating a memory block using the free() function allocated using the calloc() function:
Input:
Output:
Explanation: In this program, we have used the free() function to de-allocate the char array allocated using the calloc() function. We have de-allocated the memory pointed by the string char pointer variable during the run-time of the program.
- Deallocating array memory blocks allocated using malloc() and calloc() using free in C:
Input:
(Input can be any number)
Output:
Explanation: In this program, we have used the free() function to deallocate the memory blocks allocated using the malloc() and calloc() functions. We have deallocated the memory pointed by arr1 and arr2 integer pointer variables during the run-time of the program.
Conclusion
- free in C is a library function present in stdlib.h header file of C language.
- free in C is used to de-allocate or free up the space allocated by the functions like malloc() and **calloc()**.
- free(ptr); takes only one argument, i.e., the pointer pointing to the memory location to be de-allocated.
- free() function in C doesn't return any value. It has a return type of void.